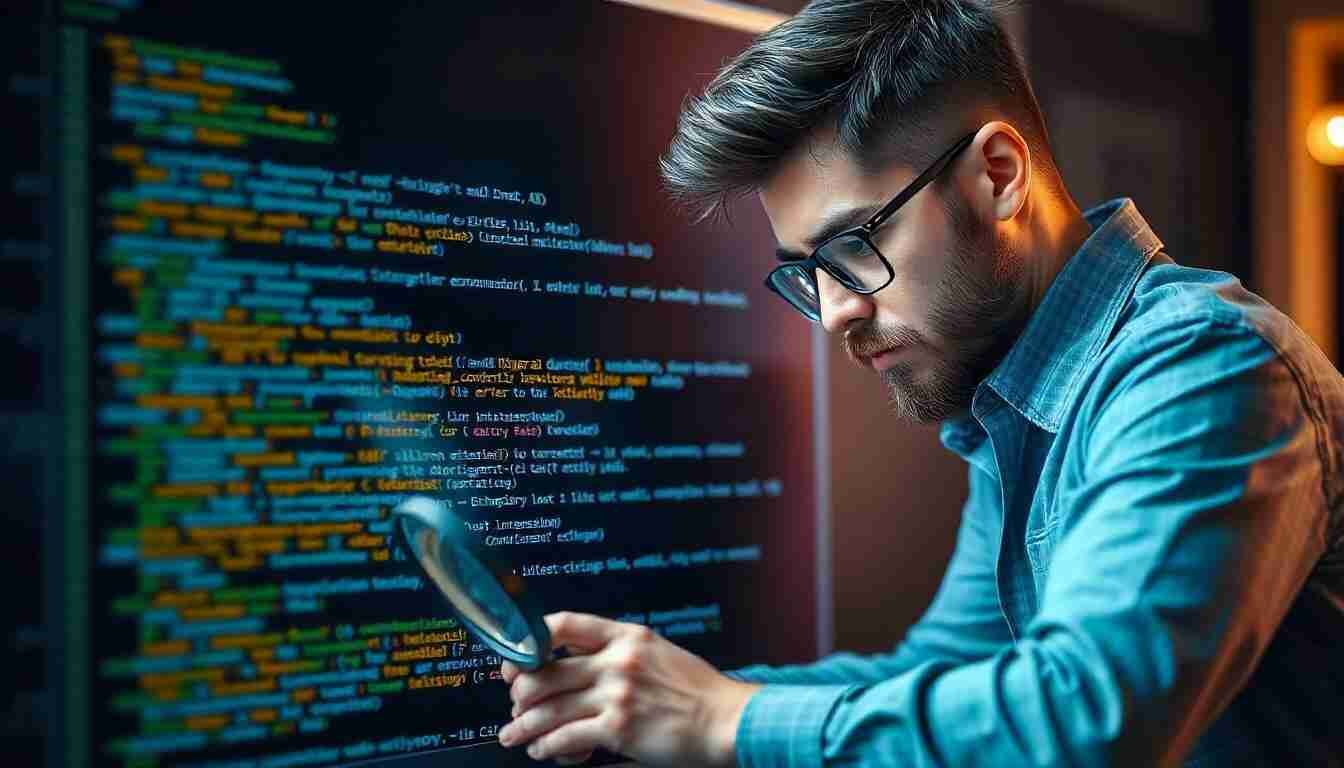
Debugging Tools for Developers: Finding and Fixing Errors
Debugging is an essential skill for any developer. It's the process of identifying and fixing errors, or bugs, in your code. These bugs can range from simple typos to complex logic errors, and they can cause your program to crash, produce unexpected results, or even compromise security.
While the process of debugging can be frustrating, it's also a rewarding experience that can help you learn and grow as a developer. Fortunately, there are many tools available to help you debug your code more efficiently.
Types of Debugging Tools
Debugging tools come in various forms, each with its own strengths and weaknesses. Some common types include:
- Debuggers: These are specialized programs that allow you to step through your code line by line, examine variables, set breakpoints, and monitor program execution. Examples include GDB, LLDB, and Visual Studio Debugger.
- Linters: These tools analyze your code for potential errors, style violations, and bad practices. They can help you catch problems early on and enforce coding standards. Popular linters include ESLint, Pylint, and RuboCop.
- Profilers: These tools help you identify performance bottlenecks in your code by measuring execution time, memory usage, and other metrics. Examples include Valgrind, gprof, and the Chrome DevTools profiler.
- Logging tools: These tools allow you to record events and messages from your program, which can be helpful for tracking down the cause of errors. Examples include Log4j, Winston, and the Python logging module.
Choosing the Right Debugging Tool
The best debugging tool for you will depend on several factors, including the programming language you're using, the type of error you're trying to fix, and your personal preferences. Here are some things to consider when choosing a tool:
- Features: Does the tool offer the features you need, such as breakpoints, watch expressions, and call stack inspection?
- Ease of use: Is the tool easy to learn and use? Does it have a user-friendly interface?
- Integration: Does the tool integrate well with your development environment and other tools?
- Community support: Is there a strong community of users who can offer help and support?
Tips for Effective Debugging
Regardless of the tools you use, there are some general tips that can help you debug your code more effectively:
- Reproduce the error: Before you start debugging, make sure you can consistently reproduce the error. This will help you narrow down the cause.
- Read the error message: Error messages often provide valuable clues about what went wrong. Pay attention to the line number and the type of error.
- Use the scientific method: Formulate a hypothesis about the cause of the error, then test it by making changes to your code and observing the results.
- Divide and conquer: Break down the problem into smaller, more manageable parts. This will make it easier to identify the source of the error.
- Don't be afraid to ask for help: If you're stuck, don't hesitate to reach out to other developers for help. There are many online communities and forums where you can get assistance.
Example: Debugging a JavaScript Error
Let's say you're working on a JavaScript web application and you encounter the following error in your browser's console:
TypeError: Cannot read property 'length' of undefined
This error message tells you that you're trying to access the length property of a variable that is undefined. To debug this error, you could use the following steps:
- Reproduce the error: Identify the steps needed to consistently trigger the error in your application.
- Inspect the code: Use your browser's developer tools to examine the line of code where the error occurs. Look for any variables that might be undefined.
- Use console.log: Add console.log statements to your code to print the values of relevant variables. This will help you track down where the variable becomes undefined.
- Set breakpoints: Use your browser's debugger to set breakpoints in your code. This will allow you to pause execution and step through the code line by line, examining the values of variables at each step.
- Test your fix: Once you've identified the cause of the error, make the necessary changes to your code and test to see if the error has been resolved.
Conclusion
Debugging is a crucial part of the software development process. By mastering debugging tools and techniques, you can become a more efficient and effective developer. Remember to choose the right tools for the job, follow best practices, and don't be afraid to seek help when needed. With patience and persistence, you can overcome any debugging challenge and deliver high-quality software.
Useful External Links